canvascv::Text Class Reference
#include <text.h>
Inheritance diagram for canvascv::Text:
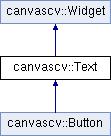
Public Member Functions | |
virtual const char * | getType () const |
getType is always implemented by derived to return the same static pointer per widget. More... | |
std::string | getText () const |
get the current text of the Text | |
void | setText (const std::string &value) |
set the current text of the Text | |
int | getFontFace () const |
get the OpenCV font used | |
void | setFontFace (int value) |
set the OpenCV font to use | |
double | getFontScale () const |
get the OpenCV scale used for te font | |
void | setFontScale (double value) |
set the OpenCV scale used for te font | |
int | getFontHeight () const |
get the calculated font height (with padding and internal font spaces) | |
void | setFontHeight (int value) |
set the font scale from a requested pixel height (with padding and internal font spaces) | |
int | getMaxWidth () const |
get the maxuimum allowed width of rows in pixels (0 means it's disabled) | |
void | setMaxWidth (int value) |
set the maxuimum allowed width of rows in pixels (0 to disable max width) | |
virtual const cv::Rect & | getRect () |
Actual size the widget is occupying due to Layout manager. | |
virtual const cv::Rect & | getMinimalRect () |
Minimal size the widget coould have occupy. | |
virtual void | recalc () |
update self so next call to 'draw' will display correctly | |
virtual void | drawFG (cv::Mat &dst) |
dst is the roi of the widget size and not the full image | |
![]() | |
Widget (const cv::Point &pos=cv::Point(0, 0)) | |
constructor | |
virtual | ~Widget () |
virtual destructor | |
void | notifyOnChange (CBWidgetState cb) |
used to register for notifications on a widget More... | |
cv::Scalar | getOutlineColor () const |
get the outline color | |
virtual void | setOutlineColor (const cv::Scalar &value) |
set the outline color | |
cv::Scalar | getFillColor () const |
get the bg color | |
virtual void | setFillColor (const cv::Scalar &value) |
set the bg color | |
bool | getVisible () const |
is the widget visible | |
virtual void | setVisible (bool value) |
set the widget visible state | |
int | getThickness () const |
get line thickness to use when drawing | |
virtual void | setThickness (int value) |
set line thickness to use when drawing | |
int | getLineType () const |
get the line type (LINE_4, LINE_8, LINE_AA) | |
virtual void | setLineType (int value) |
set the line type (LINE_4, LINE_8, LINE_AA) | |
uchar | getAlpha () const |
get the alpha value used for the widge background [0,255] => [transparent,opaque] | |
void | setAlpha (uchar value) |
set the alpha value used for the widge background [0,255] => [transparent,opaque] | |
Anchor | getLayoutAnchor () const |
getLayoutAnchor returns the anchor for using in the Layout we're in More... | |
void | setLayoutAnchor (const Anchor &value) |
setLayoutAnchor sets the anchor for using in the Layout we're in More... | |
Anchor | getFlowAnchor () const |
getFlowAnchor get internal widget alignment and direction of growth More... | |
void | setFlowAnchor (const Anchor &value) |
setFlowAnchor set internal widget alignment and direction of growth More... | |
int | getId () |
Widgets have a unique id per instance. | |
virtual const std::string & | getStatusMsg () const |
getStatusMsg More... | |
void | setStatusMsg (const std::string &value) |
setStatusMsg More... | |
const cv::Point & | getLocation () const |
get widget position in Canvas | |
virtual void | setLocation (const cv::Point &value) |
set widget position in Canvas | |
virtual void | translate (const cv::Point &translation) |
move the widget | |
bool | getStretchX () const |
get if the Layout we're in should stretch us in the X direction | |
void | setStretchX (bool value) |
set if the Layout we're in should stretch us in the X direction (and calls setStretchXToParent(false)) | |
bool | getStretchY () const |
get if the Layout we're in should stretch us in the Y direction | |
void | setStretchY (bool value) |
set if the Layout we're in should stretch us in the Y direction (and calls setStretchYToParent(false)) | |
cv::Scalar | getSelectColor () const |
get the color to use when a widget is selected | |
virtual void | setSelectColor (const cv::Scalar &value) |
set the color to use when a widget is selected | |
std::shared_ptr< Widget > | rmvFromLayout () |
get rid of the widget | |
bool | getIsSelectable () const |
get if the widget should appear as selected when the mouse is over it | |
void | setIsSelectable (bool value) |
set if the widget should appear as selected when the mouse is over it | |
int | getForcedWidth () const |
get the forced width for this widget | |
void | setForcedWidth (int value) |
set the forced width for this widget (and calls setStretchX/setStretchXToParent(false)) | |
int | getForcedHeight () const |
get the forced height for this widget | |
void | setForcedHeight (int value) |
set the forced height for this widget (and calls setStretchY/setStretchYToParent(false)) | |
void | update () |
removes 'dirty' state and invokes the derived 'recalc/recalcCompound' | |
bool | isRemoved () const |
returns true if the widget has no layout and false if it does | |
bool | getStretchXToParent () const |
get if this widget streches to parent layout width | |
void | setStretchXToParent (bool value) |
set if this widget streches to parent layout width (and calls setStretchX(false)) | |
bool | getStretchYToParent () const |
get if this widget streches to parent layout height | |
void | setStretchYToParent (bool value) |
set if this widget streches to parent layout height (and calls setStretchY(false)) | |
Static Public Member Functions | |
static std::shared_ptr< Text > | create (Layout &layout, const cv::Point &pos, const std::string &text="", Anchor flowAnchor=TOP, Anchor layoutAnchor=TOP) |
create a Text widget More... | |
static std::shared_ptr< Text > | create (Layout &layout, const std::string &text="", Anchor flowAnchor=TOP, Anchor layoutAnchor=TOP) |
a convinient version to the above without the 'pos' argument | |
Additional Inherited Members | |
![]() | |
typedef std::function< void(Widget *)> | CBWidget |
signature of a callback which only gets the widget | |
typedef std::function< void(Widget *, State)> | CBWidgetState |
signature of a callback which gets the State | |
typedef std::function< void(Widget *, int)> | CBUserSelection |
signature of a callback which gets a user selection | |
![]() | |
void | setStateChangesBG () |
widgets like buttons change bg on mouse events | |
void | allocateBG (const cv::Size &size) |
invokes Theme::allocateBG() | |
void | flatWidget () |
delegate to Theme | |
void | raisedWidget () |
delegate to Theme | |
void | sunkenWidget () |
delegate to Theme | |
void | selectedWidget () |
delegate to Theme | |
virtual void | renderOn (cv::Mat &dst) |
render the widget to dst More... | |
void | callDrawFG (bool preAllocateMat=true) |
helper method which delgates to drawFG for derived | |
bool | setDirty () |
setDirty More... | |
virtual bool | isAtPos (const cv::Point &pos) |
isAtPos More... | |
Detailed Description
Displaying text on the OpenCV Window with/without a hilighting background.
- Examples:
- example_add_theme.cpp.
Member Function Documentation
|
static |
- Parameters
-
layout widgets are placed in layouts Canvas/VFrame/HFrame/... pos location in the Layout (Layouts can ignore that) text what to write (accepts newline) flowAnchor affects the widget behavior - TOP/BOTTOM - text appears below or above pos
- RIGHT/CENTER/LEFT is row alignment for multirows
layoutAnchor affects the layout in the Layout it belongs to - TOP/CENTER/BOTTOM is relevat to a HorizontalLayout/HFrame (unless setStretchY() is used)
- RIGHT/CENTER/LEFT is relevat to a VerticalLayout/VFrame (unless setStretchX() is used)
- Returns
- a smart pointer copy of the object kept in the Layout
- Examples:
- example_shapes_widgets.cpp.
|
virtual |
- Returns
- const char * pointer to string with widget type name
Implements canvascv::Widget.
Reimplemented in canvascv::Button.
The documentation for this class was generated from the following file:
- CanvasCV-doxygen/src/canvascv/widgets/text.h
Generated by
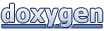