The Canvas class is the entry point into CanvasCV. More...
#include <canvas.h>
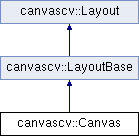
Public Types | |
typedef std::function< void(Shape *)> | CBCanvasShape |
notification on shapes create/modify/delete from this Canvas instance | |
Public Member Functions | |
Canvas (const std::string &winNameVal, cv::Size sizeVal=cv::Size()) | |
Canvas. More... | |
~Canvas () | |
release all shapes and widgets. Shape callbacks are invoked doe delete. | |
void | redrawOn (const cv::Mat &src, cv::Mat &dst) |
redrawOn draws the shapes on dst More... | |
void | redrawOn (cv::Mat &dst) |
redrawOn More... | |
void | setImage (const cv::Mat &img) |
setImage More... | |
bool | onMousePress (const cv::Point &pos) |
You should delegate OpenCV mouse callback events to this method - returns true if did something at pos. | |
void | onMouseRelease (const cv::Point &pos) |
You should delegate OpenCV mouse callback events to this method. | |
void | onMouseMove (const cv::Point &pos) |
You should delegate OpenCV mouse callback events to this method. | |
std::shared_ptr< Shape > | createShape (std::string type, const cv::Point &pos=cv::Point(0, 0)) |
createShape More... | |
template<class T > | |
std::shared_ptr< T > | createShape (const cv::Point &pos=cv::Point(0, 0)) |
createShape More... | |
std::string | getShapeType () const |
get default shape type to draw More... | |
void | setShapeType (std::string value) |
set default shape type to draw (could be "") More... | |
void | deleteActive () |
delete shape currenty selected | |
void | deleteShape (const std::shared_ptr< Shape > &shape) |
delete specific shape | |
void | deleteWidget (const std::shared_ptr< Widget > &widget) |
delete specific widget | |
void | notifyOnShapeCreate (CBCanvasShape cb) |
used to register for notifications on shape creation More... | |
void | notifyOnShapeModify (CBCanvasShape cb) |
used to register for notifications on shape modification (actually when it is deselected) More... | |
void | notifyOnShapeDelete (CBCanvasShape cb) |
used to register for notifications on shape deletion More... | |
void | clearShapes () |
clear all shapes from Canvas | |
void | clearWidgets () |
clear all widgets from Canvas | |
template<class T > | |
void | getShapes (std::list< std::shared_ptr< T >> &result) |
getShapes of a specific type More... | |
std::shared_ptr< Shape > | getShape (int id) |
getShape More... | |
void | getShapes (const cv::Point &pos, std::list< std::shared_ptr< Shape >> &result) |
getShapes More... | |
void | disableScreenText () |
disable the top left text area for manual user messages | |
void | disableStatusMsg () |
disable the bottom left text area for auto status messages | |
void | enableScreenText (cv::Scalar color=Colors::Black, cv::Scalar bgColor=Colors::LightGray, double scale=Consts::DEFAULT_FONT_SCALE, int thickness=Consts::DEFAULT_FONT_THICKNESS, uchar alpha=80, int fontFace=Consts::DEFAULT_FONT) |
enableScreenText enables the top left text area for manual user messages More... | |
void | enableStatusMsg (cv::Scalar color=Colors::Orange, cv::Scalar bgColor=Colors::LightGray, double scale=Consts::DEFAULT_FONT_SCALE, int thickness=Consts::DEFAULT_FONT_THICKNESS, uchar alpha=80, int fontFace=Consts::DEFAULT_FONT) |
enableStatusMsg enables the bottom left text area for auto status messages More... | |
void | setDefaultStatusMsg (const std::string &msg) |
set the default status message (active widget and shapes will override it) | |
std::string | getDefaultStatusMsg () const |
get the default status message (active widget and shapes will override it) | |
void | setScreenText (const std::string &msg) |
manually set the screen text message. It remains until disabled or changed. | |
void | setSize (const cv::Size &value) |
set the Canvas size to match the cv::Mat we'll use in redrawOn; | |
cv::Size | getSize () |
get the Canvas size | |
virtual void | addWidget (const std::shared_ptr< Widget > &widget) |
adds the widget to this Layout after removing it from it's previous layout | |
virtual std::shared_ptr< Widget > | rmvWidget (const std::shared_ptr< Widget > &widget) |
rmvWidget More... | |
bool | getOn () const |
is redrawOn() on/off? | |
void | setOn (bool value) |
redrawOn will do nothing if value is 'false' | |
void | writeShapesToFile (const std::string &filepath) const |
write all the shapes currently in the Canvas to a file | |
void | readShapesFromFile (const std::string &filepath) |
load all the from a file into the canvas (removing all current shapes in the process) | |
void | setMouseCallback () |
utility method to handle mouse events on the associated window | |
void | imshow (InputArray mat) |
utility method which uses the winName encapsulated in Canvas | |
int | waitKeyEx (int delay=0) |
waitKeyEx More... | |
void | applyTheme (bool applyToCanvasText=false) |
applyTheme More... | |
void | setDirty () |
while waiting for events the Canvas is redrawn only if it is dirty | |
Static Public Member Functions | |
static void | fatal (string errorMsg, int exitStatus) |
fatal More... | |
Additional Inherited Members |
Detailed Description
This is conceptually a canvas layer on top of your frame.
- It can be turned on and off.
- It can show your messages on screen with a semi transparent background.
- You can handle mouse and keyboard events:
- To create/edit/delete shapes on screen for user selections and landmark configuration.
- To handle widgets you put on the screen for user input.
Constructor & Destructor Documentation
canvascv::Canvas::Canvas | ( | const std::string & | winNameVal, |
cv::Size | sizeVal = cv::Size() |
||
) |
This class is associated with an OpenCV window of a certain size.
- Parameters
-
winNameVal is the name of the OpenCV window (it doesn't have to exist yet) sizeVal is the size of the OpenCV window
- See also
- setSize()
Member Function Documentation
void canvascv::Canvas::applyTheme | ( | bool | applyToCanvasText = false | ) |
apply the current theme to all existing widgets and shapes in the canvas
- Parameters
-
applyToCanvasText should be usually false (make it true to affect Status&UserText of Canvas).
std::shared_ptr<Shape> canvascv::Canvas::createShape | ( | std::string | type, |
const cv::Point & | pos = cv::Point(0, 0) |
||
) |
Create shape by name on the canvas directly from code (instead of by the user using the mouse)
- Parameters
-
type name of the Shape pos shape position
- Returns
- This method will return a shared_ptr<T> instance, which you don't have to keep since another one is kept by the Canvas in which the shape is placed. Never use delete on a Shape pointer.
- Examples:
- example_shapes_widgets.cpp.
std::shared_ptr< T > canvascv::Canvas::createShape | ( | const cv::Point & | pos = cv::Point(0,0) | ) |
Create shape by type on the canvas directly from code (instead of by the user using the mouse)
- Parameters
-
pos shape position
void canvascv::Canvas::enableScreenText | ( | cv::Scalar | color = Colors::Black , |
cv::Scalar | bgColor = Colors::LightGray , |
||
double | scale = Consts::DEFAULT_FONT_SCALE , |
||
int | thickness = Consts::DEFAULT_FONT_THICKNESS , |
||
uchar | alpha = 80 , |
||
int | fontFace = Consts::DEFAULT_FONT |
||
) |
During enable of this feature you can overide some default values. It is safe to call this method again just to change display settings.
- Parameters
-
color is font color bgColor is rect bg color scale is font scale thickness is font thickness alpha is the alpha value of the rect bg [0,255] => [transparent,opaque] fontFace is the OpenCV fonr to use
- Examples:
- example_checkboxes.cpp, example_radiobuttons.cpp, and example_shapes_widgets.cpp.
void canvascv::Canvas::enableStatusMsg | ( | cv::Scalar | color = Colors::Orange , |
cv::Scalar | bgColor = Colors::LightGray , |
||
double | scale = Consts::DEFAULT_FONT_SCALE , |
||
int | thickness = Consts::DEFAULT_FONT_THICKNESS , |
||
uchar | alpha = 80 , |
||
int | fontFace = Consts::DEFAULT_FONT |
||
) |
During enable of this feature you can overide some default values. It is safe to call this method again just to change display settings.
- Parameters
-
color is font color bgColor is rect bg color scale is font scale thickness is font thickness alpha is the alpha value of the rect bg [0,255] => [transparent,opaque] fontFace is the OpenCV fonr to use
- Examples:
- example_shapes_widgets.cpp.
|
static |
A more elegant way for your app to exit on failures.
- A dedicated opencv window with a MsgBox wil show your message.
- The error code will be used with _Exit()
- The errorMsg will also be written to the standard error
- Parameters
-
errorMsg will be displayed to the user exitStatus will be used with _Exit()
std::shared_ptr<Shape> canvascv::Canvas::getShape | ( | int | id | ) |
- Parameters
-
id
- Returns
- shape with requested id
void canvascv::Canvas::getShapes | ( | std::list< std::shared_ptr< T >> & | result | ) |
- Parameters
-
result will contain alll shapes of the T on return
- Note
- these are internal shapes used in the canvas, so changing them affects what is drawn on the Canvas.
void canvascv::Canvas::getShapes | ( | const cv::Point & | pos, |
std::list< std::shared_ptr< Shape >> & | result | ||
) |
- Parameters
-
pos is position to search for shapes result is all the shapes at pos
|
inline |
- Returns
- returns the default current shape to create on mouse press
void canvascv::Canvas::notifyOnShapeCreate | ( | CBCanvasShape | cb | ) |
Multiple registrations are allowed.
- Parameters
-
cb to invoke on shape creation
- Examples:
- example_shapes_widgets.cpp.
void canvascv::Canvas::notifyOnShapeDelete | ( | CBCanvasShape | cb | ) |
Multiple registrations are allowed.
- Parameters
-
cb to invoke on shape deletion
void canvascv::Canvas::notifyOnShapeModify | ( | CBCanvasShape | cb | ) |
Multiple registrations are allowed.
- Parameters
-
cb to invoke on shape modification
void canvascv::Canvas::redrawOn | ( | const cv::Mat & | src, |
cv::Mat & | dst | ||
) |
Draws src with shapes and widgets onto dst. src is upgraded to 3 channels if it has 1 channel.
- Parameters
-
src can be also dst, in which case it is drawn on. src is BGR/BGRA/GRAY. dst if different than src, then src is cloned to it and drawn on.
- See also
- setImage
- Examples:
- example_shapes_widgets.cpp.
void canvascv::Canvas::redrawOn | ( | cv::Mat & | dst | ) |
A utility method that uses latest Mat used as 'src' in the redrawOn above. This methos is also used internally in waitKeyEx(0)
- Parameters
-
dst will be used as output.
- See also
- waitKeyEx
|
virtual |
- Parameters
-
widget will be removed from this Layout
- Returns
- filled shared_ptr to removed widget or empty if not found
- Note
- Widgets must be in layouts to be displayed correctly.
Implements canvascv::Layout.
- Examples:
- example_shapes_widgets.cpp.
void canvascv::Canvas::setImage | ( | const cv::Mat & | img | ) |
sets the internal 'latestFrameSrc' used for GUI updates while waitKeyEx(0) is used, or with redrawOn(cv::Mat&)
- Parameters
-
img will be used to setSize and update internal latestFrameSrc
|
inline |
- Parameters
-
value will be the default current shape to create on mouse press
- Examples:
- example_linecrossing.cpp, example_shapes.cpp, and example_shapes_widgets.cpp.
int canvascv::Canvas::waitKeyEx | ( | int | delay = 0 | ) |
utility method to handle key strokes - you get the keystroke if a widget/shape didn't consume it.
- Parameters
-
delay in milliseconds. If delay is 0, then the latest image used as input will be the background for internal updates. If you want to change it from a callback, then use setImage.
- Returns
- the key press or -1 if the timeout reached
- See also
- setImage
- Note
- When using widgets with callback on the same image, the delay should be 0. If you're changing frames or using only the polling API of the widgets, then specify a delay of your choice.
- Examples:
- example_shapes_widgets.cpp.
The documentation for this class was generated from the following file:
- CanvasCV-doxygen/src/canvascv/canvas.h
Generated by
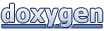