text.h
int getMaxWidth() const
get the maxuimum allowed width of rows in pixels (0 means it's disabled)
virtual void drawFG(cv::Mat &dst)
dst is the roi of the widget size and not the full image
static std::shared_ptr< Text > create(Layout &layout, const cv::Point &pos, const std::string &text="", Anchor flowAnchor=TOP, Anchor layoutAnchor=TOP)
create a Text widget
virtual void recalc()
update self so next call to 'draw' will display correctly
virtual const cv::Rect & getMinimalRect()
Minimal size the widget coould have occupy.
void setFontHeight(int value)
set the font scale from a requested pixel height (with padding and internal font spaces) ...
virtual const cv::Rect & getRect()
Actual size the widget is occupying due to Layout manager.
void setMaxWidth(int value)
set the maxuimum allowed width of rows in pixels (0 to disable max width)
int getFontHeight() const
get the calculated font height (with padding and internal font spaces)
virtual const char * getType() const
getType is always implemented by derived to return the same static pointer per widget.
Generated by
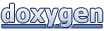