example_radiobuttons.cpp
This is an example of how to use the RadioButtons Widget.
#include "canvascv/canvas.h"
// These are used to create widgets
#include "canvascv/widgets/radiobuttons.h"
#include <iostream>
#include <iterator>
#include <opencv2/highgui.hpp>
using namespace std;
using namespace cv;
using namespace canvascv;
int main(int argc, char **argv)
{
--argc;
++argv;
Mat image;
if (argc)
{
Mat orig = imread(argv[0]);
if (orig.empty())
{
}
if (orig.cols > 1024)
{
double ratio = 1024. / orig.cols;
cv::resize(orig, image, Size(), ratio, ratio);
}
else
{
image = orig;
}
}
else
{
Canvas::fatal("Must get a path to an image as a parameter" , -1);
}
c.enableScreenText();
c.setScreenText(CCV_STR("User selected option '" << index << "': '" << rb->getTextAt(index) << "'\n"));
switch (index)
{
case 0:
break;
case 1:
break;
case 2:
break;
}
};
"Blue", // index 0
"Green", // index 1
"Red", // index 2
"Exit" // index 3
}, 0, cb,
Point(image.cols / 2., image.rows / 2.));
radioButtons->setOutlineColor(Colors::Blue);
namedWindow("Canvas", WINDOW_AUTOSIZE);
c.setMouseCallback(); // optional for mouse usage see also (example_selectbox.cpp)
int key = 0;
int delay = 1000/25; // we use a delay for polling the radioButtons
Mat out; // keeping it out of the loop is a little more efficient
while (radioButtons->getSelection() != 3 && key != 'q')
{
c.redrawOn(image, out);
imshow("Canvas", out);
key = c.waitKeyEx(delay); // GUI and callbacks happen here
}
destroyAllWindows();
return 0;
}
Generated by
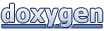