canvascv::LineCrossing Class Reference
The LineCrossing class. More...
#include <linecrossing.h>
Inheritance diagram for canvascv::LineCrossing:
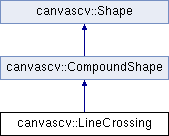
Public Member Functions | |
int | getDirection () const |
-1 or 1 | |
const std::string & | getName () const |
gets the text from the attached TextBox | |
void | setName (const std::string &value) const |
sets the text in the attached TextBox | |
TextBox * | getTextBox () |
getter to internal TextBox | |
Line * | getLine () |
getter to internal Line | |
Arrow * | getArrow () |
getter to internal Arrow | |
bool | wasCrossed (const Point &pt) const |
wasCrossed More... | |
int | isCrossedBySegment (const Point &lineStart, const Point &lineEnd) const |
isCrossedBy More... | |
virtual bool | isAtPos (const cv::Point &pos) |
returns true if shape is at pos, false otherwise | |
virtual std::list< Handle * > | getConnectionTargets () |
getConnectionTargets More... | |
virtual const char * | getType () const |
getType is always implemented by derived to return the same static pointer per shape. More... | |
![]() | |
virtual void | setOutlineColor (const cv::Scalar &value) |
set the outline color | |
virtual void | setFillColor (const cv::Scalar &value) |
set the fill color (fill color is not very useful for shapes right now) | |
virtual void | setThickness (int value) |
set line thickness to use when drawing | |
virtual void | setLineType (int value) |
set the line type (LINE_4, LINE_8, LINE_AA) | |
virtual void | setLocked (bool value) |
set the shape lock state (can/can't be moved/edited) | |
virtual void | setVisible (bool value) |
set the shape visible state | |
virtual std::shared_ptr< Shape > | getShape (int id) |
getShape More... | |
![]() | |
Shape () | |
constructor | |
Shape (const Shape &other) | |
copy constructor | |
virtual | ~Shape () |
virtual destructor | |
void | notifyOnEvent (CBPerShape cb) |
used to register for notifications on shape More... | |
cv::Scalar | getOutlineColor () const |
get the outline color | |
cv::Scalar | getFillColor () const |
get the fill color (fill color is not very useful for shapes right now) | |
bool | getLocked () const |
is the shape locked (can't be moved/edited) | |
bool | getVisible () const |
is the shape visible | |
int | getThickness () const |
get line thickness to use when drawing | |
int | getLineType () const |
get the line type (LINE_4, LINE_8, LINE_AA) | |
int | getId () |
return a unique id for this shape | |
Protected Member Functions | |
virtual void | draw (cv::Mat &canvas) |
draw shape on the canvas More... | |
virtual bool | mousePressed (const cv::Point &pos, bool onCreate=false) |
mousePressed More... | |
![]() | |
virtual bool | mouseMoved (const cv::Point &pos) |
mouseMoved More... | |
virtual bool | mouseReleased (const cv::Point &pos) |
mouseReleased More... | |
virtual bool | keyPressed (int &key) |
keyPressed will be called by Canvas for active shapes More... | |
virtual void | lostFocus () |
lostFocus is called by Canvas if we're in it and just became non-active | |
![]() | |
void | drawHelper (cv::Mat &canvas, Shape *other) |
helper method for non compund shapes to draw their members | |
Additional Inherited Members | |
![]() | |
typedef std::function< void(Shape *, Event)> | CBPerShape |
signature of a callback which gets the Event | |
Detailed Description
- Helps us know when something we tracked passed over a line.
- We also need to know if it passed in one direction or another.
- Examples:
- example_linecrossing.cpp, and example_shapes_widgets.cpp.
Member Function Documentation
|
protectedvirtual |
- Parameters
-
canvas
Reimplemented from canvascv::CompoundShape.
|
virtual |
Return a list of Handles this shape allows to connect to from other shapes (mainly for ShapesConnector)
- Returns
- list of Handle pointers we ShapesConnector can use to connect
Implements canvascv::Shape.
|
virtual |
- Returns
- const char * pointer to string with shape type name
Implements canvascv::Shape.
int canvascv::LineCrossing::isCrossedBySegment | ( | const Point & | lineStart, |
const Point & | lineEnd | ||
) | const |
Use cross product and predefined direction to tell if a given line path, starting at lineStart and ending at lineEnd is crossing this specific line segment .
- Parameters
-
lineStart the start of the line to check against (path origin Point). lineEnd the end of the line to check against (path latest Point).
- Returns
- 0 if the segments are not crossing
- 1 if the segment crossed according to direction
- -1 if the segment crossed against the direction
- Examples:
- example_linecrossing.cpp.
|
protectedvirtual |
- Parameters
-
pos onCreate is true if this is the mouse press which cerated this shape
- Returns
- true for keep in focus, false for leave focus
Reimplemented from canvascv::CompoundShape.
bool canvascv::LineCrossing::wasCrossed | ( | const Point & | pt | ) | const |
Use cross product and predefined direction to tell if a given point is accross the endless line represented by this segment.
- Parameters
-
pt is the point we're going to examine
- Returns
- bool if line is on the other side of the line according to the direction arrow
The documentation for this class was generated from the following file:
- CanvasCV-doxygen/src/canvascv/shapes/linecrossing.h
Generated by
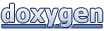