canvascv::TextBox Class Reference
#include <textbox.h>
Inheritance diagram for canvascv::TextBox:
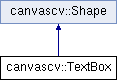
Public Member Functions | |
const std::string & | getText () const |
get the displayed text | |
void | setText (const std::string &value) |
set the displayed text | |
void | setTL (const cv::Point &value) |
set position (top left corner) | |
int | getFontFace () const |
get the font used | |
void | setFontFace (int value) |
set the font used | |
double | getFontScale () const |
get the scale of the used font | |
void | setFontScale (double value) |
set the scale of the used font | |
int | getFontThickness () const |
get the thickness of the font | |
void | setFontThickness (int value) |
set the thickness of the font | |
cv::Scalar | getFontColor () const |
get the font color | |
void | setFontColor (const cv::Scalar &value) |
set the font color | |
virtual bool | isAtPos (const cv::Point &pos) |
returns true if shape is at pos, false otherwise | |
virtual std::list< Handle * > | getConnectionTargets () |
getConnectionTargets More... | |
virtual std::shared_ptr< Shape > | getShape (int id) |
getShape More... | |
virtual const char * | getType () const |
getType is always implemented by derived to return the same static pointer per shape. More... | |
![]() | |
Shape () | |
constructor | |
Shape (const Shape &other) | |
copy constructor | |
virtual | ~Shape () |
virtual destructor | |
void | notifyOnEvent (CBPerShape cb) |
used to register for notifications on shape More... | |
cv::Scalar | getOutlineColor () const |
get the outline color | |
virtual void | setOutlineColor (const cv::Scalar &value) |
set the outline color | |
cv::Scalar | getFillColor () const |
get the fill color (fill color is not very useful for shapes right now) | |
virtual void | setFillColor (const cv::Scalar &value) |
set the fill color (fill color is not very useful for shapes right now) | |
bool | getLocked () const |
is the shape locked (can't be moved/edited) | |
virtual void | setLocked (bool value) |
set the shape lock state (can/can't be moved/edited) | |
bool | getVisible () const |
is the shape visible | |
virtual void | setVisible (bool value) |
set the shape visible state | |
int | getThickness () const |
get line thickness to use when drawing | |
virtual void | setThickness (int value) |
set line thickness to use when drawing | |
int | getLineType () const |
get the line type (LINE_4, LINE_8, LINE_AA) | |
virtual void | setLineType (int value) |
set the line type (LINE_4, LINE_8, LINE_AA) | |
int | getId () |
return a unique id for this shape | |
Protected Member Functions | |
virtual void | draw (cv::Mat &canvas) |
draw shape on the canvas More... | |
virtual bool | mousePressed (const cv::Point &pos, bool onCreate=false) |
mousePressed More... | |
virtual bool | mouseMoved (const cv::Point &pos) |
mouseMoved More... | |
virtual bool | mouseReleased (const cv::Point &pos) |
mouseReleased More... | |
virtual bool | keyPressed (int &key) |
keyPressed will be called by Canvas for active shapes More... | |
virtual void | lostFocus () |
lostFocus is called by Canvas if we're in it and just became non-active | |
![]() | |
void | drawHelper (cv::Mat &canvas, Shape *other) |
helper method for non compund shapes to draw their members | |
Additional Inherited Members | |
![]() | |
typedef std::function< void(Shape *, Event)> | CBPerShape |
signature of a callback which gets the Event | |
Detailed Description
Allows you to draw a line by mouse or from code
- Examples:
- example_shapes_widgets.cpp.
Member Function Documentation
|
protectedvirtual |
- Parameters
-
canvas
Implements canvascv::Shape.
|
virtual |
Return a list of Handles this shape allows to connect to from other shapes (mainly for ShapesConnector)
- Returns
- list of Handle pointers we ShapesConnector can use to connect
Implements canvascv::Shape.
|
virtual |
Get internal shapes, which Canvas doesn't know of.
- Parameters
-
id
- Returns
- internal sub shape with requested id
Reimplemented from canvascv::Shape.
|
virtual |
- Returns
- const char * pointer to string with shape type name
Implements canvascv::Shape.
|
protectedvirtual |
- Parameters
-
key was pressed. You must set it to -1 if you consumed it.
- Returns
- true if we want to stay in focus and false otherwise
Reimplemented from canvascv::Shape.
|
protectedvirtual |
- Was a mouse moved over this shape?
- If shape is during edit, then these are the mouse position.
- Parameters
-
pos
- Returns
- true if a mouse moved over this shape, or it is during edit. false otherwise.
Implements canvascv::Shape.
|
protectedvirtual |
- Parameters
-
pos onCreate is true if this is the mouse press which cerated this shape
- Returns
- true for keep in focus, false for leave focus
Implements canvascv::Shape.
|
protectedvirtual |
The documentation for this class was generated from the following file:
- CanvasCV-doxygen/src/canvascv/shapes/textbox.h
Generated by
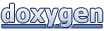