widget.h
433 // These write and read functions must be defined for the serialization in cv::FileStorage to work
Anchor getLayoutAnchor() const
getLayoutAnchor returns the anchor for using in the Layout we're in
Definition: widget.h:138
void setStateChangesBG()
widgets like buttons change bg on mouse events
uchar getAlpha() const
get the alpha value used for the widge background [0,255] => [transparent,opaque] ...
void setForcedHeight(int value)
set the forced height for this widget (and calls setStretchY/setStretchYToParent(false)) ...
void setIsSelectable(bool value)
set if the widget should appear as selected when the mouse is over it
void setForcedWidth(int value)
set the forced width for this widget (and calls setStretchX/setStretchXToParent(false)) ...
virtual void setSelectColor(const cv::Scalar &value)
set the color to use when a widget is selected
bool getIsSelectable() const
get if the widget should appear as selected when the mouse is over it
bool isRemoved() const
returns true if the widget has no layout and false if it does
cv::Scalar getSelectColor() const
get the color to use when a widget is selected
virtual void recalc()=0
update self so next call to 'draw' will display correctly
virtual void setLineType(int value)
set the line type (LINE_4, LINE_8, LINE_AA)
std::function< void(Widget *, State)> CBWidgetState
signature of a callback which gets the State
Definition: widget.h:45
virtual const cv::Rect & getRect()=0
Actual size the widget is occupying due to Layout manager.
void setFlowAnchor(const Anchor &value)
setFlowAnchor set internal widget alignment and direction of growth
virtual void drawFG(cv::Mat &dst)=0
dst is the roi of the widget size and not the full image
void setLayoutAnchor(const Anchor &value)
setLayoutAnchor sets the anchor for using in the Layout we're in
virtual void setThickness(int value)
set line thickness to use when drawing
Anchor getFlowAnchor() const
getFlowAnchor get internal widget alignment and direction of growth
Definition: widget.h:157
bool getStretchYToParent() const
get if this widget streches to parent layout height
void update()
removes 'dirty' state and invokes the derived 'recalc/recalcCompound'
void setStretchX(bool value)
set if the Layout we're in should stretch us in the X direction (and calls setStretchXToParent(false)...
virtual const char * getType() const =0
getType is always implemented by derived to return the same static pointer per widget.
bool getStretchY() const
get if the Layout we're in should stretch us in the Y direction
std::function< void(Widget *, int)> CBUserSelection
signature of a callback which gets a user selection
Definition: widget.h:48
void setStretchXToParent(bool value)
set if this widget streches to parent layout width (and calls setStretchX(false)) ...
void setStretchY(bool value)
set if the Layout we're in should stretch us in the Y direction (and calls setStretchYToParent(false)...
void callDrawFG(bool preAllocateMat=true)
helper method which delgates to drawFG for derived
bool getStretchX() const
get if the Layout we're in should stretch us in the X direction
void setStretchYToParent(bool value)
set if this widget streches to parent layout height (and calls setStretchY(false)) ...
virtual void setLocation(const cv::Point &value)
set widget position in Canvas
void notifyOnChange(CBWidgetState cb)
used to register for notifications on a widget
virtual const cv::Rect & getMinimalRect()=0
Minimal size the widget coould have occupy.
virtual void setOutlineColor(const cv::Scalar &value)
set the outline color
std::function< void(Widget *)> CBWidget
signature of a callback which only gets the widget
Definition: widget.h:42
bool getStretchXToParent() const
get if this widget streches to parent layout width
void setAlpha(uchar value)
set the alpha value used for the widge background [0,255] => [transparent,opaque] ...
Generated by
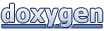