widgetfactory.h
37 static std::shared_ptr<Widget> newWidget(std::string type, Layout &layoutVal, const cv::Point &pos);
105 #define CCV_REGISTER_WIDGET(X) static bool regWidget##X = canvascv::WidgetFactoryT<X>::addType(#X)
static std::shared_ptr< Widget > newWidget(std::string type, Layout &layoutVal, const cv::Point &pos)
newWidget
static void applyCurrentTheme(Shape *shape)
used automatically by the ShapeFactory when creating a shape
static std::shared_ptr< T > newWidget(Layout &layoutVal, const cv::Point &pos=cv::Point(0, 0))
newWidget
Definition: widgetfactory.h:95
static bool addType(std::string name)
addType adds type 'T' under name 'name'
Definition: widgetfactory.h:83
virtual void addWidget(const std::shared_ptr< Widget > &widget)=0
adds the widget to this Layout after removing it from it's previous layout
Generated by
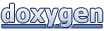