Table of Contents
Giving the user multiple options and collecting them is common to canvascv::CheckBoxes, canvascv::RadioButtons and canvascv::SelectionBox.
We going to take a look at using the CheckBoxes now. The concept is similar to RadioButtons and the SelectionBox. In the tutorial about layout managers we'll see an example frame with several of them together.
CheckBoxes with a callback
This is the normal and preffered way of working with widgets.
The CheckBox alows you to register a canvascv::Widget::CBUserSelection callback, which will be called on user altering the widget selections.
You get the widget and an index to the item that changed. It is then up to you to inspect the widget at that index (or other indexes).
See this code example, and read the notes after it:
Notes:
- CCV_STR lets you create a string as you would write into a stream.
- This tutorial is using C++11 lambda expressions as callbacks, but anything which has the "void(Widget*,int)" signature will work.
- Note the alternate way of displaying images
- use canvascv::Canvas::setImage() for the image you're showing.
- use canvascv::Canvas::waitKeyEx() without arguments to refresh widgets and handle internal events with your image as the background.
- Here is a possible outcome of this code:
CheckBoxes with polling
Using polling might serve you right for some reason...
Instead of using a callback, you can check the values on each iteration.
This is not the recommended way.
See this code and read the notes after it:
Notes:
- When polling is used we need all these stages in the main loop -
- canvascv::Canvas::redrawOn()
- canvascv::Canvas::imshow()
- canvascv::Canvas::waitKeyEx() with a non zero delay
- Here is a possible outcome of this code:
That's all for this tutorial
Generated by
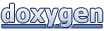