msgbox.h
static int createModal(const std::string &title, const std::string &msg, std::vector< std::string > buttonNames={"Ok"}, Widget::CBUserSelection cbUserSelection=Widget::CBUserSelection())
createModal
static std::shared_ptr< MsgBox > create(Canvas &canvas, const std::string &msg, std::vector< std::string > buttonNames={"Ok"}, Widget::CBUserSelection cbUserSelection=Widget::CBUserSelection(), const cv::Point &pos=cv::Point(-1,-1))
create a message box widget which is closed automatically
std::function< void(Widget *, int)> CBUserSelection
signature of a callback which gets a user selection
Definition: widget.h:48
virtual const char * getType() const
getType is always implemented by derived to return the same static pointer per widget.
Generated by
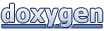