matwidget.h
virtual const cv::Rect & getMinimalRect()
Minimal size the widget coould have occupy.
const cv::Mat & getMat() const
get the FG Mat which is displayed by the widget
virtual void recalc()
update self so next call to 'draw' will display correctly
virtual const char * getType() const
getType is always implemented by derived to return the same static pointer per widget.
virtual void drawFG(cv::Mat &dst)
dst is the roi of the widget size and not the full image
virtual const cv::Rect & getRect()
Actual size the widget is occupying due to Layout manager.
static std::shared_ptr< MatWidget > create(Layout &layout, cv::Mat mat=cv::Mat(), const cv::Point &pos=cv::Point(0, 0))
create a MatWidget widget
Generated by
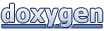