shapesconnector.h
void connectHead(Shape &shape, Handle &handle)
Connect 'shape' with it's Handle 'handle' to our own head Handle.
void reconnect()
called after read of Canvas from file, assuming internals are filled, but not connected ...
virtual const char * getType() const
getType is always implemented by derived to return the same static pointer per shape.
void setSpacing(int value)
set the spacing of the dotted connecting line
void disconnectHead()
disconnect our own head Handle from any previously connected shape
virtual bool mousePressed(const cv::Point &pos, bool onCreate=false)
mousePressed
virtual bool mouseReleased(const cv::Point &pos)
mouseReleased
int getSpacing() const
get the spacing of the dotted connecting line
virtual std::list< Handle * > getConnectionTargets()
getConnectionTargets
void disconnectTail()
disconnect our own tail Handle from any previously connected shape
void disconnectShape(int id)
disconnect a previouslt connected shape with an 'id'
Shape * getHeadShape()
get the shape connected to our head Handle
int getTailShapeId()
get the id of the shape connected to our tail Handle
Definition: shapesconnector.h:40
Shape * getTailShape()
get the shape connected to our tail Handle
void connectTail(Shape &shape, Handle &handle)
Connect 'shape' with it's Handle 'handle' to our own tail Handle.
int getHeadShapeId()
get the id of the shape connected to our head Handle
Definition: shapesconnector.h:46
Generated by
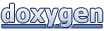