autolayout.h
int getMaxWidgetWidth()
get the maximum width from all contained widgets
void setPadding(int value)
setPadding set number of pixels to pad from Layout rect during layout
T * at(int index)
return a widget at index 'i' (or 0 if type is wrong ot 'i' is too big)
Definition: autolayout.h:102
void setWrap(bool value)
set if the layout will wrap widgets past forcedWidth / forcedHeight
virtual const char * getType() const =0
getType is always implemented by derived to return the same static pointer per widget.
bool getWrap() const
get if the layout will wrap widgets past forcedWidth / forcedHeight
int getMaxWidgetHeight()
get the maximum height from all contained widgets
virtual void addWidget(const std::shared_ptr< Widget > &widget)
adds the widget to this Layout after removing it from it's previous layout
virtual std::shared_ptr< Widget > rmvWidget(const std::shared_ptr< Widget > &widget)
rmvWidget
int getPadding() const
getPadding get number of pixels to pad from Layout rect during layout
Generated by
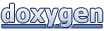